2021-11-19KMP算法KMP 算法(Knuth-Morris-Pratt 算法)是一个著名的字符串匹配算法,效率很高,但是比较难以理解
详情 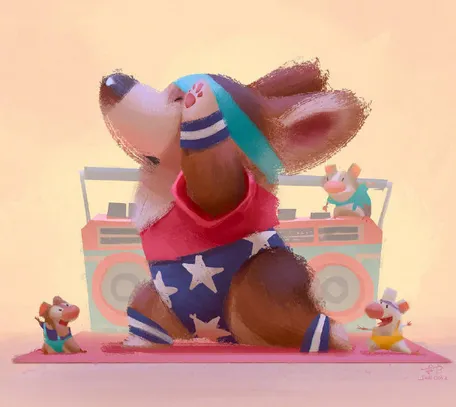
2021-11-16双指针算法双指针算法的核心思想:找单调性,优化暴力枚举。找单调性,找单调性,让指针不用回退可以一往无前~
详情 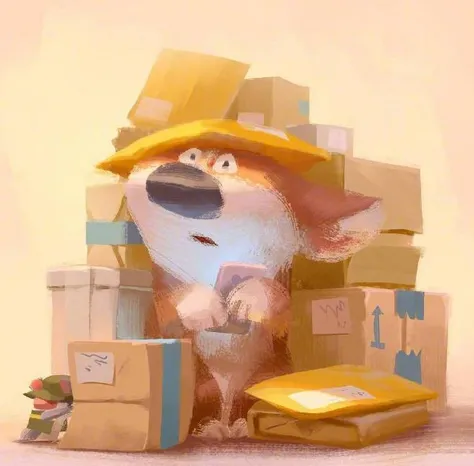
2021-11-19栈与队列把栈想象成以一个有底的桶,先进后出;把队列想象成一个两通的管道,先进先出
详情 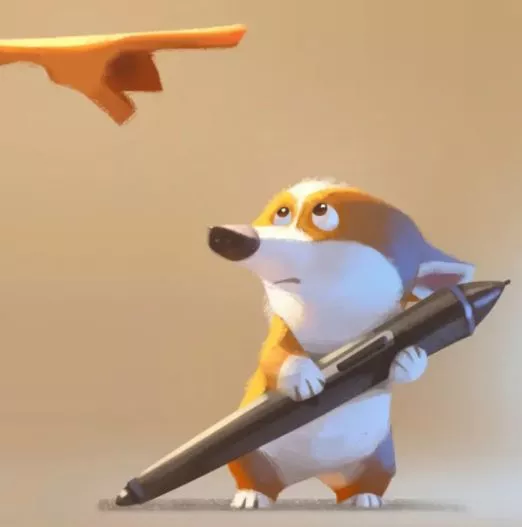
2021-11-13排序算法快排其实利用了双指针算法的思想,先调整区间再递归处理,这很好
详情 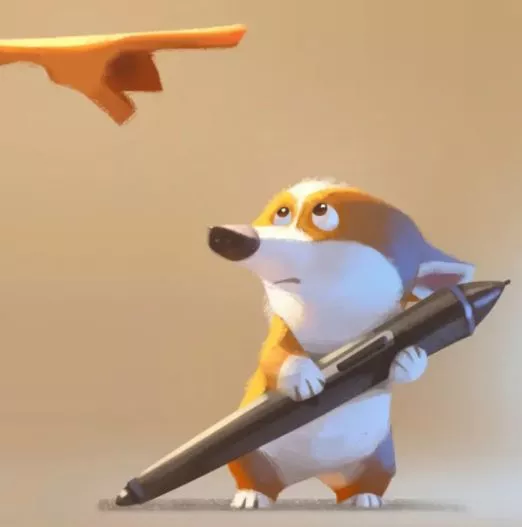
2021-11-14二分查找二分查找,相当食用的查找方法,不仅在算法题中,在生活中也可以用到哦
详情 ')}for(var elist="undefined".split(","),cpage=location.pathname,epage="all",flag=0,i=0;i